Programming Apps
ATM App Code
#include <iostream>
#include <iomanip>
void showBalance (double balance);
double deposit();
double withdraw(double balance);
int main(){
double balance =0;
int choice = 0;
do{
std::cout << "**********\n";
std::cout << "Enter your choice: \n";
std::cout << "**********\n";
std::cout << "1. Show Balance\n";
std::cout << "2. Deposit Money\n";
std::cout << "3. Withdraw Money\n";
std::cout << "4. Exit\n";
std::cin >> choice;
std::cin.clear();
fflush(stdin);
switch(choice){
case 1: showBalance (balance);
break;
case 2: balance+=deposit();
showBalance (balance);
break;
case 3: balance -=withdraw(balance);
showBalance (balance);
break;
case 4: std::cout <<"Thanks for visting!\n";
break;
default: std::cout << "Invalid choice!\n";
}
}
while (choice !=4);
}
void showBalance(double balance){
std::cout << "Your balance is: $"<< std::setprecision(2) << std::fixed << balance <<'\n';
}
double deposit(){
double amount = 0;
std::cout <<"Enter amount to be deposited: ";
std::cin>> amount;
if(amount> 0){
return amount;}
else{
std::cout <<"Thats not a valid amount\n";
return 0;
}
}
double withdraw(double balance){
double amount =0;
std::cout<< "Enter amount to be withdrawn: ";
std::cin>> amount;
if(amount> balance){
std::cout <<"insufficient fund\n";
return 0;
}
else if(amount<0){
std::cout<<"That aint valid:\n";
return 0;
}
else{
return amount;}
}
This code runs like an ATM machine. With 3 categories: Balance, Withdraw and Deposit. The user is able to access all options and exit when done.
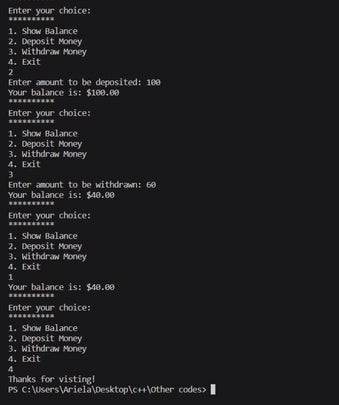
Rock, Paper Scissors Game
#include <iostream>
#include <ctime>
char getUserChoice ();
char getComputerChoice();
void showChoice(char choice);
void chooseWinner(char player, char computer);
int main(){
char player;
char computer;
player = getUserChoice();
std::cout << "You choice: ";
showChoice(player);
computer = getComputerChoice();
std::cout << "Computer's choice: ";
showChoice(computer);
chooseWinner(player, computer);
}
char getUserChoice (){
char player;
std::cout <<"Rock-Paper-Scissors Game!\n";
do{
std::cout <<"Choose one of the following\n";
std::cout <<"***************\n";
std::cout <<"'r' for rock\n";
std::cout <<"'s' for scissors\n";
std::cout <<"'p' for papaer\n";
std::cin >> player;
}
while(player !='r'&& player !='s' && player !='p');
return player;
}
char getComputerChoice(){
srand(time(0));
int num =rand() % 3 + 1;
switch(num){
case 1: return 'r';
case 2: return 's';
case 3: return 'p';
}
}
void showChoice(char choice){
switch(choice){
case 'r' : std::cout<< "Rock\n";
break;
case 's' : std::cout<< "Scissors\n";
break;
case 'p' : std::cout<< "Paper\n";
break;
}
}
void chooseWinner(char player, char computer){
switch(player){
case 'r': if(computer == 'r'){
std::cout <<"It's a tie!\n";
}
else if( computer == 'p'){
std::cout << "You lose!\n";
}
else{
std::cout << "You win!\n";
}
break;
case 's': if(computer == 's'){
std::cout <<"It's a tie!\n";
}
else if( computer == 'r'){
std::cout << "You lose!\n";
}
else{
std::cout << "You win!\n";
}
break;
case 'p': if(computer == 'p'){
std::cout <<"It's a tie!\n";
}
else if( computer == 's'){
std::cout << "You lose!\n";
}
else{
std::cout << "You win!\n";
}
break;
}
}
This Game is automated against the computer. User choose an option and either Wins or Loses.
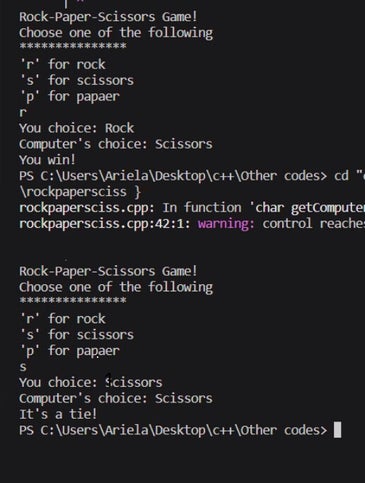
Weather Statistics Analysis
Weather.cpp
#include "Weather.h"
#include <string>
using namespace std;
Weather::Weather()
{ month_Name = "";
high_Temp = 0;
low_Temp = 0;
total_Rainfall = 0.0;
}
Weather::Weather(string month, int highTemp, int lowTemp, double totalRain) {
month_Name = month;
high_Temp = highTemp;
low_Temp = lowTemp;
total_Rainfall = totalRain;
}
Weather::~Weather()
{
}
Weather.h
#ifndef WEATHER_H
#define WEATHER_H
#pragma once
using namespace std;
class Weather
{
private:
string month_Name;
int high_Temp;
int low_Temp;
double total_Rainfall;
public:
Weather();
Weather(string month, int highTemp, int lowTemp, double totalRain);
~Weather() {};
string getMonth_Name() {
return month_Name;
}
int getHigh_Temperature() {
return high_Temp;
}
int getLow_Temperature() {
return low_Temp;
}
double getTotal_Rainfall() {
return total_Rainfall;
}
};
#endif
Source
// Joseph-Odede Ariela 400479484
#include <iostream>
#include <fstream>
#include <string>
#include "Weather.h"
using namespace std;
int main() {
Weather weatherData[12];
ifstream weatherFile("weather.txt");
if (!weatherFile.is_open()) {
cout << "Error opening file" << endl;
return 1;
}
for (int i = 0; i < 12; i++) {
string month;
int highTemp, lowTemp;
double totalRain;
weatherFile >> month >> highTemp >> lowTemp >> totalRain;
weatherData[i] = Weather(month, highTemp, lowTemp, totalRain);
}
weatherFile.close();
double total_MonthlyRainfall = 0;
double total_YearlyRainfall = 0;
int highest_Temp = -999;
int lowest_Temp = 999;
string highest_TempMonth;
string lowest_TempMonth;
for (int i = 0; i < 12; i++) {
total_MonthlyRainfall += weatherData[i].getTotal_Rainfall();
total_YearlyRainfall += weatherData[i].getTotal_Rainfall();
if (weatherData[i].getHigh_Temperature() > highest_Temp) {
highest_Temp = weatherData[i].getHigh_Temperature();
highest_TempMonth = weatherData[i].getMonth_Name();
}
if (weatherData[i].getLow_Temperature() < lowest_Temp) {
lowest_Temp = weatherData[i].getLow_Temperature();
lowest_TempMonth = weatherData[i].getMonth_Name();
}
}
cout << "Average monthly rainfall: " << total_MonthlyRainfall / 12 << endl;
cout << "Total rainfall for the year: " << total_YearlyRainfall << endl;
cout << "Highest temperature: " << highest_Temp << " (" << highest_TempMonth << ")" << endl;
cout << "Lowest temperature: " << lowest_Temp << " (" << lowest_TempMonth << ")" << endl;
return 0;
}
This program gets input from the user on all the monthly rainfall & Temperature. therefore calculates the average rainfall, adds the Total Rainfall, compare the months for highest and lowest temperature.
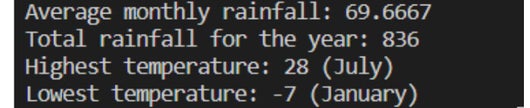
Car Repair Workshop
Transmission.h
#ifndef TRANSMISSION_H
#define TRANSMISSION_H
#include "Repair.h"
#include <iomanip>
#include <string>
#include <iostream>
#include <cstdlib>
#include <ctime>
using namespace std;
class Transmission
{
private:
string Part_Name;
double Part_Cost;
double Labour_Cost;
int Labour_Hours;
protected:
public:
Transmission();
void partcost();
string GetName() { return Part_Name;}
double GetCost() {return Part_Cost;}
double GetLabourCost() { return Labour_Cost;}
int GetLabour() {return Labour_Hours;}
};
#endif
.cpp
#include "Transmission.h"
Transmission::Transmission() {
Part_Name = "";
Part_Cost = 0;
Labour_Cost = 0;
Labour_Hours = 0;
}
void Transmission::partcost() {
int num = rand() % 3 + 1;
if (num == 1) {
Part_Name = "Repair Transmission and fill Fluid";
Part_Cost = 2500.00;
Labour_Cost = 500.00;
Labour_Hours = 24;
}
if (num == 2) {
Part_Name = "Replace Transmission Fluid";
Part_Cost = 95.00;
Labour_Cost = 80.00;
Labour_Hours = 2;
}
if (num == 3) {
Part_Name = "Both the Transmission and Fluid are in good condition";
Part_Cost = 0.00;
Labour_Cost = 10.00;
Labour_Hours = 1;
}
};
Tires.h
#ifndef TIRES_H
#define TIRES_H
#include <iostream>
#include <iomanip>
#include <string>
#include <cmath>
#include <cstdlib>
#include <time.h>
#include <limits.h>
#include <fstream>
using namespace std;
class Tires
{
private:
string Part_Name;
double Part_Cost;
double Labour_Cost;
int Labour_Hours;
protected:
public:
Tires();
void partcost();
string GetName() { return Part_Name; };
double GetCost() { return Part_Cost; };
int GetLabour() { return Labour_Hours; };
double GetLabourCost() { return Labour_Cost; };
};
#endif
.cpp
#include "Tires.h"
#include <iostream>
#include <iomanip>
#include <string>
#include <cmath>
#include <cstdlib>
#include <time.h>
#include <limits.h>
#include <fstream>
using namespace std;
Tires::Tires()
{
Part_Name = "";
Part_Cost = 0;
Labour_Cost = 0;
Labour_Hours = 0;
}
void Tires::partcost()
{
int number = rand() % 6 + 1;
if (number == 1)
{
Part_Name = "The tires need air in them";
Part_Cost = 0.0;
Labour_Cost = 9.99;
Labour_Hours = 1;
}
if (number == 2)
{
Part_Name = "The tires need an alignment";
Part_Cost = 199.99;
Labour_Cost = 49.99;
Labour_Hours = 2;
}
if (number == 3)
{
Part_Name = "The tires need to be balanced";
Part_Cost = 39.99;
Labour_Cost = 24.99;
Labour_Hours = 1;
}
if (number == 4)
{
Part_Name = "The tires need to be rotated";
Part_Cost = 14.99;
Labour_Cost = 9.99;
Labour_Hours = 1;
}
if (number == 5)
{
Part_Name = "The vehicle needs new tires";
Part_Cost = 999.99;
Labour_Cost = 99.99;
Labour_Hours = 2;
}
if (number == 6)
{
Part_Name = "The vehicles wheels are ready to hit the raod";
Part_Cost = 0.0;
Labour_Cost = 29.99;
Labour_Hours = 1;
}
Paint_and_Coatings.h
#ifndef PAINT_AND_COATINGS_H
#define PAINT_AND_COATINGS_H
#include <iomanip>
#include <string>
#include <iostream>
#include <cstdlib>
#include <ctime>
using namespace std;
class Paint_and_Coatings
{
private:
string Part_Name;
double Part_Cost;
double Labour_Cost;
int Labour_Hours;
protected:
public:
Paint_and_Coatings();
void partcost();
string GetName() { return Part_Name; }
double GetCost() { return Part_Cost; }
double GetLabourCost() { return Labour_Cost; }
int GetLabour() { return Labour_Hours; }
};
#endif
.cpp
#include "Paint_and_Coatings.h"
Paint_and_Coatings::Paint_and_Coatings() {
Part_Name = "";
Part_Cost = 0;
Labour_Cost = 0;
Labour_Hours = 0;
}
void Paint_and_Coatings::partcost() {
int num = rand() % 8 + 1;
if (num == 1) {
Part_Name = "Vinyl with Glossy Clear Coating";
Part_Cost = 3500.00;
Labour_Cost = 1250.00;
Labour_Hours = 0;
}
if (num == 2) {
Part_Name = "Vinyl with Matte Clear Coating";
Part_Cost = 4000.00;
Labour_Cost = 4000.00;
Labour_Hours = 2;
}
if (num == 3) {
Part_Name = "Vinyl with Glossy Premium Coating ";
Part_Cost = 4000.00;
Labour_Cost = 1250.00;
Labour_Hours = 1;
}
if (num == 4) {
Part_Name = "Vinyl with Matte Premium Coating";
Part_Cost = 4500.00;
Labour_Cost = 1250.00;
Labour_Hours = 0;
}
if (num == 5) {
Part_Name = "Paint with Glossy Clear Coating";
Part_Cost = 6000.00;
Labour_Cost = 2000.00;
Labour_Hours = 0;
}
if (num == 6) {
Part_Name = "Paint with Matte Clear Coating";
Part_Cost = 6500.00;
Labour_Cost = .00;
Labour_Hours = 0;
}
if (num == 7) {
Part_Name = "Paint with Glossy Premium Coating";
Part_Cost = 6500.00;
Labour_Cost = 2000.00;
Labour_Hours = 0;
}
if (num == 8) {
Part_Name = "Paint with Matte Premium Coating";
Part_Cost = 7000.00;
Labour_Cost = 2000.00;
Labour_Hours = 0;
}
};
Lighting.h
#ifndef LIGHTING_H
#define LIGHTING_H
#include <iostream>
#include <iomanip>
#include <string>
#include <cmath>
#include <cstdlib>
#include <time.h>
#include <limits.h>
#include <fstream>
using namespace std;
class Lighting
{
private:
string Part_Name;
double Part_Cost;
double Labour_Cost;
int Labour_Hours;
protected:
public:
Lighting();
void partcost();
string GetName() { return Part_Name; };
double GetCost() { return Part_Cost; };
int GetLabour() { return Labour_Hours; };
double GetLabourCost() { return Labour_Cost; };
};
#endif
.cpp
#include "Lighting.h"
#include <iostream>
#include <iomanip>
#include <string>
#include <cmath>
#include <cstdlib>
#include <time.h>
#include <limits.h>
#include <fstream>
using namespace std;
Lighting::Lighting()
{
Part_Name = "";
Part_Cost = 0;
Labour_Cost = 0;
Labour_Hours = 0;
}
void Lighting::partcost()
{
int number = rand() % 9 + 1;<span data-ccp-props="{"20134
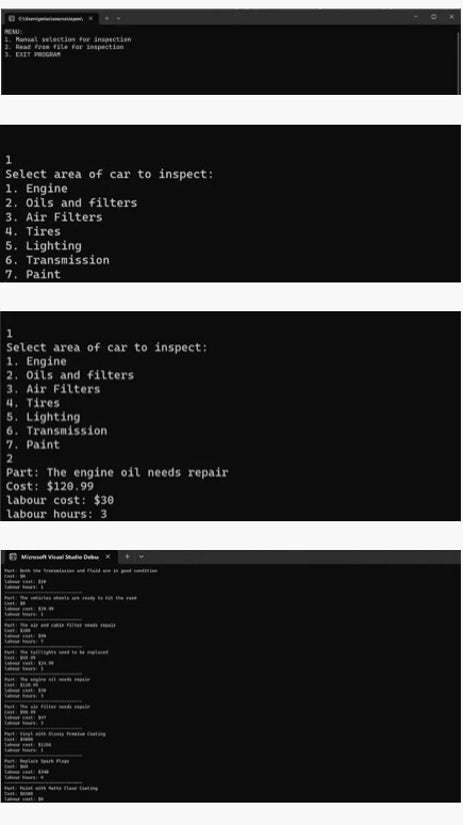
Company Management App
Source.cpp
#include "Teamleader.h"
#include <iostream>
using namespace std;
int main()
{
TeamLeader d("Mark", "124586", "2021-07-21", 2, 30.0, 100, 70, 50);
cout << d << endl;
}
Employee.h
#ifndef EMPLOYEE_H
#define EMPLOYEE_H
#include <iostream>
#include <string>
#pragma once
using namespace std;
class Employee
{
private:
string name;
string employ_num;
string hire_date;
public:
Employee();
Employee(string, string, string);
Employee(const Employee&);
void setName(string n) { name = n; }
void setEmploy_Num(string e) { employ_num = e; }
void setHire_Date(string h) { hire_date = h; }
string getName() { return name; }
string getEmploy_Num() { return employ_num; }
string getHire_Date() { return hire_date; }
void print() const;
};
#endif
Employee.cpp
#include "Employee.h"
Employee::Employee() {
name = " ";
employ_num = " ";
hire_date = " ";
}
Employee::Employee(string n, string e, string h) {
name = n;
employ_num = e;
hire_date = h;
}
Employee::Employee(const Employee& e) {
name = e.name;
employ_num = e.employ_num;
hire_date = e.hire_date;
}
void Employee::print() const {
std::cout << " Employee Name: " << name << std::endl;
std::cout << " Employee Number: " << employ_num << std::endl;
std::cout << " Employee Hire Date: " << hire_date << std::endl;
}
ProductionWorker.h
#ifndef PRODUCTIONWORKER_H
#define PRODUCTIONWORKER_H
#include "Employee.h"
#pragma once
class ProductionWorker {
protected:
int shift;
double pay_rate;
Employee info;
public:
ProductionWorker();
ProductionWorker(int, double, Employee);
void setPay_Rate(double p) {
pay_rate = p;
}
double getPay_Rate() { return pay_rate; }
void print()const;
};
#endif
ProductionWorker.cpp
#include "ProductionWorker.h"
ProductionWorker::ProductionWorker() {
pay_rate = 30;
info = Employee();
}
ProductionWorker::ProductionWorker(int s,double p, Employee i) {
shift = s;
pay_rate = p;
info = i;
}
void ProductionWorker::print()const {
std::cout << " Pay Rate: " << pay_rate << std::endl;
}
Teamleader.h
#ifndef TEAMLEADER_H
#define TEAMLEADER_H
#include "ProductionWorker.h"
#pragma once
class TeamLeader : public ProductionWorker {
private:
int bonus_amount;
int train_hours;
int hours_attended;
public:
TeamLeader();
TeamLeader(int, int, int);
TeamLeader(int, int, int,int, double, Employee);
TeamLeader(string, string, string,int, double, int, int, int);
void setBonus_Amount(int b) {
bonus_amount = b;
}
void setTrain_hours(int t) {
train_hours = t;
}
void setHours_attended(int h) {
hours_attended = h;
}
int getBonus_Amount() {
return bonus_amount;
}
int getTrain_hours() {
return train_hours;
}
int getHours_attended() {
return hours_attended;
}
void print() const;
friend std::ostream& operator << (std::ostream&, TeamLeader&);
};
#endif
Teamleader.cpp
#include "TeamLeader.h"
TeamLeader::TeamLeader() {
bonus_amount = 0;
train_hours = 0;
hours_attended = 0;
}
TeamLeader::TeamLeader(int b, int t, int a) {
bonus_amount = b;
train_hours = t;
hours_attended = a;
}
TeamLeader::TeamLeader(int b, int t, int a, int s,double p, Employee i) : ProductionWorker(s,p, i) {
bonus_amount = b;
train_hours = t;
hours_attended = a;
}
TeamLeader::TeamLeader(string n, string e, string h,int s,double p, int b, int t, int a) :
ProductionWorker( s,p, Employee( n, e,h)) {
bonus_amount = b;
train_hours = t;
hours_attended = a;
}
void TeamLeader::print() const {
info.print();
ProductionWorker::print();
std::cout << "Bonus amount: " << bonus_amount << std::endl;
std::cout << " Required Training hours: " << train_hours << std::endl;
std::cout << "Training Hours attended:" << hours_attended << std::endl;
}
std::ostream& operator<< (std::ostream& out, TeamLeader& r) {
out <<" Employee Name: " << r.info.getName() << std::endl;
out <<" Employee Number: " << r.info.getEmploy_Num() << std::endl;
out <<" Employee Hire date: " << r.info.getHire_Date() << std::endl;
if (r.shift == 1) {
out << " Shift: Day shift\n";
}
else {
out << " Shift: Night shift\n";
}
out << " Pay Rate: " << r.pay_rate << std::endl;
out << "Bonus amount: " << r.bonus_amount << std::endl;
out << " Required Training hours: " << r.train_hours << std::endl;
out << "Training Hours attended:" << r.hours_attended << std::endl;
out << std::endl;
return out;
}
This program manages employee details in the system using classes In OOP
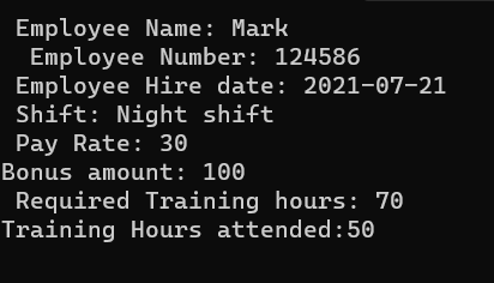
Create Your Own Website With Webador